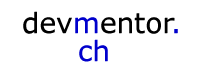 |
|
home |
10. Abend Übung
|
Übungsaufgabe 1
|
|
Schreibe eine Funktion mit
dem Namen "SwapLongs". Diese Funktion soll zwei ihr übergebene
Parameter vom Datentyp long tauschen ( = swap) , so dass folgendes
Programm die gewünschte Ausgabe erzeugt. Überlege Dir auch was
die Funktion für einen Rückgabewert haben muss. |
|
int main()
{
long a = 10;
long b = 20;
cout << a << endl;
cout << b << endl;
SwapLongs(a, b);
cout << a << endl;
cout << b << endl;
return 0;
}
gewünschte Ausgabe : 10 20 20 10
|
Lösung 1
|
|
#include <iostream>
using namespace std;
// SwapLongs mit Referenzen
void SwapLongs(long& x, long& y)
{
long temp = x;
x = y;
y = temp;
}
int main()
{
long a = 10;
long b = 20;
cout << a << endl;
cout << b << endl;
SwapLongs(a, b);
cout << a << endl;
cout << b << endl;
return 0;
}
|
Übungsaufgabe 2
|
|
Schreibe nun eine zweite Funktion
"SwapLongs". Diesmal soll die Funktion aber mit Zeigern
funktionieren ! Schreibe also die Funktion mit long* als Übergabeparameter.
Schreibe auch gleich das main aus der Übung von vorhin so um, dass
das Beispiel kompiliert und das richtige ausgibt ! |
Lösung
|
|
#include <iostream>
using namespace std;
// SwapLongs mit Zeigern
void SwapLongs(long* px, long* py)
{
long temp = *px;
*px = *py;
*py = temp;
}
int main()
{
long a = 10;
long b = 20;
cout << a << endl;
cout << b << endl;
// Hier mit address of operator &
SwapLongs(&a, &b);
cout << a << endl;
cout << b << endl;
return 0;
}
|
Übungsaufgabe 3
|
|
Schreibe mit Hilfe der SwapLong-Funktion
eine Funktion, die vier long Parameter sortiert, s dass folgendes main die
gewünschte Ausgabe erzeugt. Verwende hierfür den Tauschsort-Algorithmus.
Hierbei werden in einer Schleife die beiden benachbarten Zahlen getauscht,
falls die zweite kleiner als die erste ist. Die Schleife wird dann abgebrochen,
wenn die Bedingung nicht mehr eintrifft und keine Zahlen mehr getauscht
wurden. Sie kann auch maximal so häufig abgearbeitet werden wie es
Elemente zu sortieren hat. |
|
int main()
{
long r = 63;
long s = 20;
long t = 11;
long u = 6;
cout << r << endl;
cout << s << endl;
cout << t << endl;
cout << u << endl;
Sort4Longs(r, s, t, u);
cout << r << endl;
cout << s << endl;
cout << t << endl;
cout << u << endl;
return 0;
}
Ausgabe :
63
20
11
6
6
11
20
63
|
Lösung
|
|
#include <iostream>
using namespace std;
void SwapLongs(long& x, long& y)
{
long temp = x;
x = y;
y = temp;
}
void Sort4Longs(long& a, long& b, long& c, long& d)
{
for(long i = 0; i < 4; ++i)
{
if(b < a) // ist b kleiner als a
{
SwapLongs(a, b);
}
if(c < b)
{
SwapLongs(b, c);
}
if(d < c)
{
SwapLongs(c, d);
}
}
}
int main()
{
long r = 63;
long s = 20;
long t = 11;
long u = 6;
cout << r << endl;
cout << s << endl;
cout << t << endl;
cout << u << endl;
Sort4Longs(r, s, t, u);
cout << r << endl;
cout << s << endl;
cout << t << endl;
cout << u << endl;
return 0;
}
|